CRM API
Overview
The CRM API is used to interact with the CRM for Jira application remotely, for example when synchronizing companies and contacts with other systems.
Base URL & Call Structure
Each CRM API call has the same structure, like the following:
YOUR_JIRA_ADRESS/plugins/servlet/crm/api?apiKey=apiKey&userName=userName&command=command
where:
apiKey – determines the identity of the caller. This parameter accepts the Key specified for the CRM app on a particular instance in CRM Administration → Integrations → Access API.
userName – determines which actions the caller can take. This parameter accepts the login of a Jira user. ⚠️ Note that Jira permissions and CRM permissions of the user are taken into account.
command – determines the required action. See the list of available commands below.
Available Commands
Action | Command Value | Description & Command Example |
---|---|---|
Get companies | getcompanies | Gets the list of companies with all their attributes. No extra parameters required. Example:
Response Example
CODE
|
Get a company | getcompanybyname | Gets a company with all its attributes by its name or ID. Requires one of the following parameters: companyId or companyName. Examples:
Response Example
CODE
|
Create a company | addcompany | Adds an entry in the list of CRM Companies. The required company attributes should be specified as additional parameters. The existing values cannot be set if the attribute is unique. Example:
Response Example
CODE
|
Update a company | editcompany | Update a company attribute value using by its id or name. Example:
Response Example
CODE
|
Delete a company | deletecompany | Delete the specified entry from the companies directory. Requires the following parameter: companyName. Example:
Response Example
CODE
|
Get contacts | getcontacts | Get the list of contacts with all their attributes. No extra parameters required. Example:
Response Example
CODE
|
Get a contact | getcontactbyname | Requires Access to main menu CRM permissions. Gets the contact with all its attributes by its name or ID. Requires one of the following parameters: contactID or contactName. Examples:
Response Example
CODE
|
Create a contact | addcontact | Adds an entry in the list of CRM Contacts. The required contact attributes should be specified as additional parameters. The existing values cannot be set if the attribute is unique. Example:
Response Example
CODE
|
Update a contact | editcontact | Requires Edit contacts permissions. Update a contact attribute value using by its id. Example:
Response Example
CODE
|
Update a contact – link to an existing Company | setcontact | Includes a contact to an existing company. Requires the following parameters: companyName/companyId and contactName/contactID Example:
Response Example
CODE
|
Delete a contact | deletecontact | Delete the specified entry from the contacts directory. Requires the following parameters: contactName. Example:
Response Example
CODE
|
Create a custom directory entry | adddictionaryvalue | Adds an entry to a speified custom directory. Requires the following parameters: dictionaryName and newvalue (becomes a name o the entry) Example:
Response Example
CODE
|
Delete a custom directory entry | removedictionaryvalue | Delete the specified entry from the specified custom directory. Requires the following parameters: dictionaryName and valuetoremove. Example:
Response Example
CODE
|
Get entries by attributes' values | searchEntities | Get CRM objects by parameters. Requires tableName and the attribute – value pairs (crm_param_1=attribute&crm_param_1_value=value) Example:
Response Example
CODE
|
Get transactions | transactionsList | Gets the list of CRM transactions with their parameters. Example:
Response Example
CODE
|
Updates a CRM Property custom field | setcrmproperties | Updates a specified field of the CRM Property type. Requires issueId (custom field ID) Example:
Response Example
CODE
|
Start synchronization process | sync | Starts synchronization of JIRA users and CRM contacts. Example:
Response Example
CODE
|
Create/Update a directory entry | updateOrCreateEntity | Creates or updates an entity in the specified directory. Requires tableName and the attribute – value pairs (crm_param_1=attribute&crm_param_1_value=value) Example:
Response Example
CODE
|
Common Errors
Invalid API key
{"success": false, "details":"Invalid apikey!"}
Caller not found
{"success": false, "details":"User username can not be found!"}
Missed parameters
{"success": false, "details":"userName, apiKey and command parameters are required!"}
Either authentification, authorization or command are not specified.
{"success": false, "details":"parameter or parameter parameters are required!"}
One or several of the required parameters are not specified.
Non-existing command
{"success": false, "details":"only sync, getcompanies, addcompany, getcompanybyname, editcompany, deletecompany, getcontacts, addcontact, getcontactbyname, editcompany, deletecontact, setcontact, unsetcontact, setcrmproperties, adddictionaryvalue, searchEntities, editcontact, transactionslist commands allowed"}
No such entry
{"success": false, "details":"entry can not be found!"}
Duplicative value
{"success": false, "details":[{"fieldCode":"fieldcode","errorMessage":"This value is already in use"},{"fieldCode":"fieldcode","errorMessage":"This value is already in use"}]}
The attribute value that should be unique already exists.
Not enough permissions
{"success": false, "details":"To access this resource user need "sample_permissions" permissions."}
Find Table Names, Attribute Codes, etc.
Table Names
The following table names are available for the standard CRM Directories:
CLIENTS
CONTACTS
PRODUCTS
TRANSACTIONS
BUDGET
As for the custom directories, you can check the table name as following:
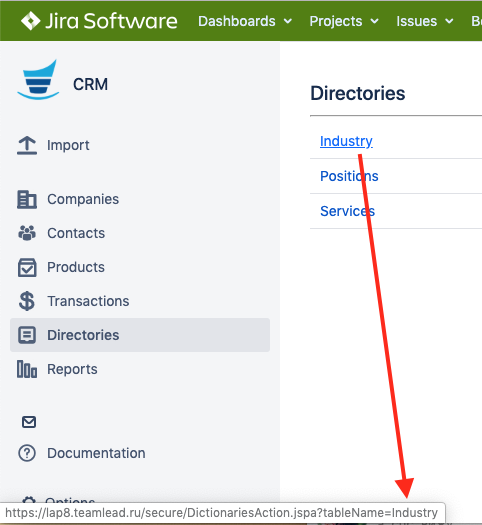
List of Table Attributes
To get the list of particular table attributes' name (no codes), perform the following call:
YOUR_JIRA_ADDRESS/rest/admin_rest/1.0/fields/TABLENAME/fieldsList
Attribute Code
To get the attributes' codes and values for the particular entry, perform the following call:
YOUR_JIRA_ADDRESS/rest/catalog-rest/1.0/catalog/getNomDetails?nomId=ENTRY_ID&tableName=TABLENAME
Entry ID
To find an entry ID open it in the full mode:
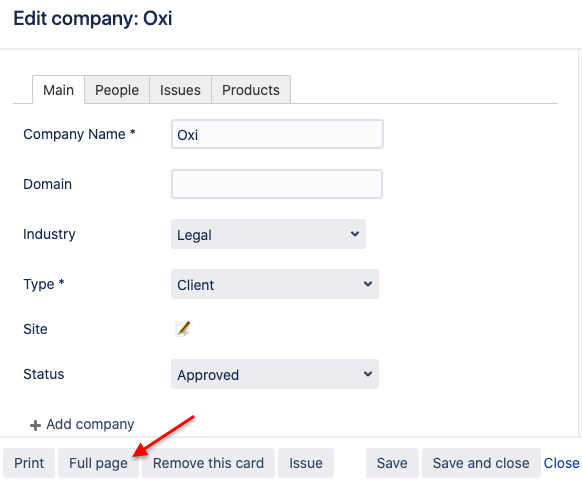
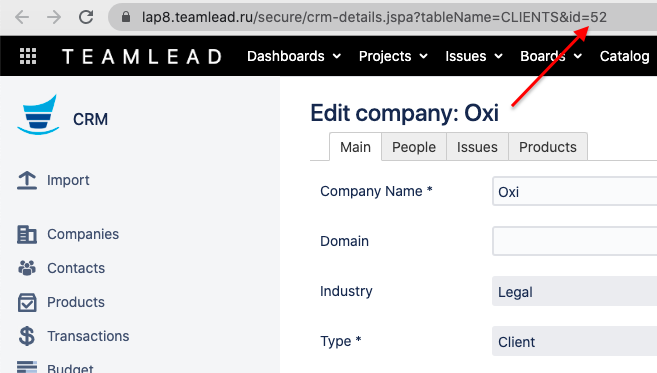